springboot项目结构:
controller:控制器层,导入service层,调用service方法;通过接受前端传过来的参数进行业务操作,再返回一个指定的路径或数据表
bean/entity/model:实体类,映射数据库中的字段
mapper/dao:放的是对数据库的操作,如CRUD
service:业务层,存放业务逻辑处理;不直接对数据库进行操作,有接口和接口实现类;提供controller层调用的方法
-
controller:控制器层,导入service层,调用service方法;通过接受前端传过来的参数进行业务操作,再返回一个指定的路径或数据表
-
bean/entity/model:实体类,映射数据库中的字段
-
mapper/dao:放的是对数据库的操作,如CRUD
-
service:业务层,存放业务逻辑处理;不直接对数据库进行操作,有接口和接口实现类;提供controller层调用的方法
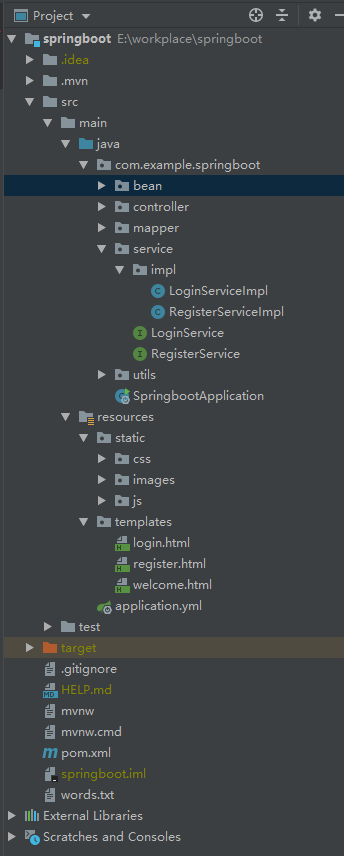
springboot模板类型
Spring Boot提供了默认配置的模板引擎主要有以下几种:
- Thymeleaf(官方推荐)
- FreeMarker
- Velocity
- Groovy
- Mustache
- JSP
模板Thymeleaf
到pom.xml文件中添加依赖
<!-- 模板引擎 Thymeleaf 依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
配置application.yml文件
spring:
thymeleaf:
prefix:
classpath: /templates/
check-template-location: true
cache: false
suffix: .html
encoding: UTF-8
mode: HTML5
servlet:
content-type: text/html
传参
@Controller
@RequestMapping("/thymeleaf")
public class ThymeleafController {
@RequestMapping("")
public ModelAndView index(){
List<User> userList = new ArrayList<User>();
User user1 = new User("solidwang", "solidwang@126.com");
User user2 = new User("jobs", "jobs@me.com");
userList.add(user1);
userList.add(user2);
ModelAndView modelAndView = new ModelAndView("/index");
modelAndView.addObject("userList", userList);
return modelAndView;
}
}
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>learn Resources</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
<div>
<h1>Thymeleaf测试</h1>
<table border="1" cellspacing="1" cellpadding="0">
<tr>
<td>姓名</td>
<td>passport</td>
</tr>
<tr th:each="user : ${userList}">
<td th:text="${user.username}">solidwang</td>
<td th:text="${user.passport}">solidwang@me.com</td>
</tr>
</table>
</div>
</body>
</html>